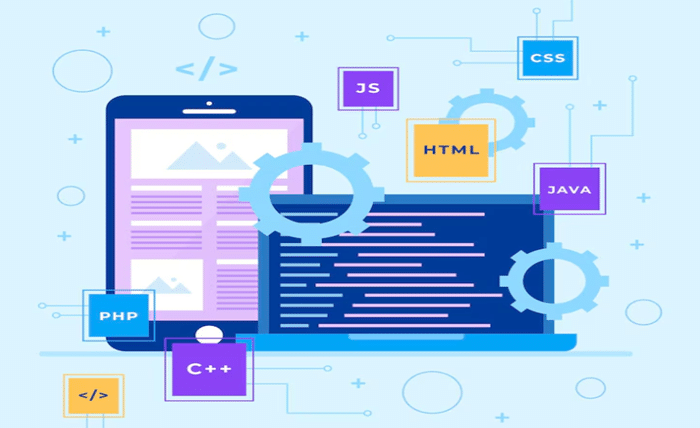
Agility and reliability are now the cornerstones of successful projects in the fast-moving world of software development. Automation testing, a linchpin of such projects, is majorly driven by Selenium Python, a popular combination within the automation testing world that offers unparalleled flexibility and efficiency. However, writing scalable, maintainable, and robust test scripts goes far beyond using basic Selenium commands and requires a structured approach with advanced techniques.
With this, we had the Page Object Model: a design pattern that Changed how we look upon organizing automation tests because it keeps page-specific elements from tearing test logic apart. Think of POM as your blueprint for a well-architected testing framework that is way easier to upkeep and adapt when applications evolve.ย
Alongside advanced locators such as XPath and CSS selectors, POM is a powerful enabler that equips testers to master complex DOMs, solve dynamic content problems, and make scripts reliable across browsers.
Understanding Page Object Model – POM
The Page Object Model, or POM, is a design pattern that suggests test logic be taken away from the page-specific logic-in turn making the automation tests maintainable and readable. POM helps to create a mapping between the UI of web pages and the test scripts. Imagine you are working on a large e-commerce application; having a clear separation of page elements, which can change frequently, from the test logic is crucial.
The Benefits of POM
- Modularity: Each page of your application can be encapsulated within its class, making it easier to manage and maintain.
- Reusability: Common functions and elements can be reused across different test cases.
- Readability: POM structures tests in a way that makes it clear what each test is verifying without diving deep into the procedural code.
- Ease of Updates: If there is a change in the UI, only the respective page object needs to be updated, minimizing the effort required across multiple test scripts.
Incorporating POM into your Selenium Python framework involves creating a class for each web page, defining methods that interact with elements on that page, and utilizing these methods in your test scripts.
Setting Up the POM Structure
Hereโs how you can structure your Selenium Python project using POM:
- Create Page Classes: Define a class for each page in your application. For example, you might have LoginPage, HomePage, and ProductPage classes.
- Identify Web Elements: Use Seleniumโs locators (like By. ID, By. XPATH) to define the web elements in each class.
- Define Methods for Interactions: Include methods in each class that allow interaction with the page elements. For instance, if you have a login page, you might include methods for entering the username and password and clicking the login button.
- Use the Page Objects in Tests: In your test scripts, you can create instances of your page classes, accessing their methods to perform actions, making tests more concise and understandable.
Advanced Locators in Selenium Python
Locators are essential in Selenium, as they identify elements on a web page to interact with during automation testing. While standard locators like ID, Name, and Class Name work well, advanced locators can be particularly useful for dynamic pages where elements may not have stable attributes.
Common Advanced Locators
- XPath: XPath allows for traversing an entire XML document. You can use both absolute and relative XPath. A robust example is using conditions to select elements based on their visibility.
- CSS Selectors: CSS selectors are faster than XPath and provide wide-ranging options for selecting elements. For example, div.classname > span#id selects a span that is a child of a div with a specific class.
- Link Text and Partial Link Text: Particularly useful for elements containing hyperlinks. Using find_element_by_link_text() lets you locate the element based on the visible text.
- JavaScript Execution: If an element cannot be located even through XPath or CSS, sometimes executing JavaScript (via driver.execute_script()) may yield results. This method can interact with hidden elements or trigger actions not available through typical Selenium commands.
Combining POM with Advanced Locators
Combining POM with advanced locators increases the robustness of your tests. For instance, a Page Object for a product page can use complex CSS selectors to identify products while keeping your test logic clean and concise. Such an approach is instrumental in applications with dynamically generated content or frequently changing interfaces.
For example, in a product page class, you can define a method like get_product_price using an advanced CSS selector that accurately targets the price element, ensuring you retrieve the correct price, even if the structure of the DOM changes slightly.
Tips for Effective Implementation
- Keep It Simple: While it may be tempting to create complex locators, aim for simple and readable patterns that everyone on your team can understand.
- Consistent Naming Conventions: Use consistent naming for methods and web elements in your POM classes. This standardization vastly increases code readability.
- Continuous Integration: Integrate your Selenium Python tests with CI/CD pipelines. Tools like Jenkins can run your tests every time a code change is made, ensuring stability.
Leveraging LambdaTest in Your POM Strategy
In today’s fast-paced development environment, ensuring your web application functions across various browsers and devices is critical. This is where LambdaTest shines. LambdaTest is a cloud-based testing platform that allows you to perform automated and manual testing across numerous browsers and versions.
LambdaTest is an AI-powered test orchestration and execution platform that lets you conduct automation testing at scale with over 3000+ real devices, browsers and OS combinations.
With LambdaTest, you can run your Selenium Python scripts across many browser and operating system combinations without going through the hassle of managing virtual machines. This cloud platform seamlessly integrates with your test framework, allowing you to run tests in parallel, thereby accelerating test execution.ย
It also supports conducting tests with real devices, which is essential for verifying mobile responsiveness. As browsers continually evolve, leveraging such a platform for cross-browser testing minimizes the risk of overlooking compatibility issues.
Current Trends in Selenium Python and Automation Testing
Given that automation testing trends continue to evolve, staying abreast of current developments is crucial. Microservices, serverless architecture, and machine learning-infused testing tools are becoming increasingly prevalent. These advancements allow for smarter testing approaches and enhance the capabilities of Selenium.
With a surge in AI-driven test automation tools, some organizations are beginning to complement their Selenium frameworks with machine learning to optimize test cases and predict failures before they happen. Furthermore, tools that integrate with Selenium, such as visual testing solutions, are gaining traction for their ability to detect UI discrepancies effectively.
Top 10 Best Practicesย
Implementing the Page Object Model (POM) and advanced locators effectively is critical to building a robust, maintainable, and scalable test automation framework. Below are some best practices to ensure success:
1. Structure Your POM Framework Effectively
- Create a Logical Folder Hierarchy
Organize your project into clear folders such as page_objects, test_cases, and utilities. This separation ensures better navigation and easier maintenance. - One Class per Page
Assign each web page its class. For example, create LoginPage for login functionality and HomePage for homepage interactions. This ensures modularity and simplicity. - Define Methods for Specific Actions
Encapsulate actions related to a specific page, such as enter_username() or click_login_button(), within the respective page class. This improves readability and test logic abstraction.
2. Use Descriptive and Consistent Naming Conventions
- Name your page object classes, methods, and locators intuitively to convey their purpose. For instance, username_input is clearer than input1.
- Adopt a consistent naming standard across your framework for ease of collaboration and onboarding.
3. Leverage Advanced Locators for Complex Pages
- XPath Optimization: Write efficient, relative XPath expressions to locate elements reliably. Avoid brittle absolute XPaths that break with minor DOM changes.
- Example: Use //div[@class=’product-title’ and contains(text(), ‘Laptop’)] instead of /html/body/div[3]/div[2]/div[1]/div.
- CSS Selectors for Speed: When performance is a concern, opt for CSS selectors over XPath. Theyโre faster and often easier to read.
- Example: Use div.product-list > span.price to locate price spans inside product lists.
- Dynamic Element Handling: Utilize contains, starts-with, or ends-with functions in XPath to handle dynamic attributes.
- Fallback to JavaScript Execution: If an element is hidden or hard to locate, use Seleniumโs JavaScript execution capabilities.
4. Embrace Modularity and Reusability
- Abstract common actions into utility functions or base classes. For example, create a BasePage class with shared methods like wait_for_element() or navigate_to_url().
- Avoid redundancy by reusing locators and methods across multiple test cases.
5. Integrate with Continuous Integration/Continuous Deployment (CI/CD)
- Configure your tests to run automatically in a CI/CD pipeline with tools like Jenkins, GitHub Actions, or GitLab CI. This ensures early detection of issues and maintains code stability.
6. Adopt Parallel and Cross-Browser Testing
- Run tests in parallel to accelerate execution time using frameworks like Pytest or integrations with Selenium Grid.
- Use tools like LambdaTest to execute tests across different browsers and operating systems. This ensures comprehensive compatibility testing and efficient test coverage.
7. Maintain Readable and Scalable Test Scripts
- Avoid hardcoding data or locators. Instead, use a data-driven approach by storing test data in external files like JSON or CSV.
- Regularly refactor your tests and locators to prevent outdated code from accumulating in your framework.
8. Implement Robust Error Handling
- Add try-except blocks in your methods to handle exceptions gracefully and capture meaningful logs or screenshots for debugging.
- Use assertions wisely to ensure test validation criteria are met.
9. Monitor and Analyze Test Results
- Integrate reporting tools like Allure or Pytest-html to generate comprehensive test reports with screenshots, logs, and metrics.
- Regularly review test results to identify flakiness, recurring failures, or performance bottlenecks.
10. Collaborate and Document
- Document your POM structure, naming conventions, and locator strategies. This ensures team alignment and helps onboard new contributors faster.
- Foster collaboration between QA and development teams to align on locator strategies and potential DOM changes.
By following these best practices, you can build a robust Selenium Python framework that not only adheres to industry standards but also adapts seamlessly to the evolving demands of your application. This approach minimizes maintenance effort, reduces test flakiness, and enhances overall automation efficiency.
Conclusion
As you advance in your testing practices with Selenium Python, embracing the Page Object Model and advanced locators will undeniably propel your automation testing efforts to new heights. By building scalable and maintainable frameworks, you set yourself up for long-term success in software development and quality assurance.
With platforms like LambdaTest facilitating comprehensive testing across browsers and devices, your automation journey becomes smoother and more efficient. So, jump into the world of Selenium Python, explore the potential of automation testing, and take your projects to new levels of reliability and functionality.
Focusing on best practices and continuous learning from the ever-changing space called ‘tech’ equips you with improved skills and builds up your quality software that will meet user expectations in this digital world.